In the next sections we’ll introduce some of the common data structures offered by Java as part of the Java Collections Framework.
This framework offers a core set of interfaces that defines the contract available to users of the different types of collections.
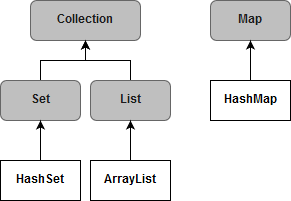
The diagram above shows the hierarchy of the interfaces (in gray background) and classes (in white background), with each subinterface or subclass providing a more specific implementation of a data structure. The diagram only covers the classes and interfaces we’ll cover in this section.
It’s important to note that all these interfaces and classes are generic, with the type parameter representing the type of element they are storing, for example a list of integer numbers can be represented as List<Integer>
.
Below is a quick summary from top-to-bottom of the interfaces in the diagram:
-
Collection: A collection is a group of elements of the same type. The collection interface provides no further details about how the objects are stored, but it provides the common behaviors across all collection types. For example, the
Collection
contract specifies methods that allow users to add/remove objects and methods to iterate over the objects in the collection. Sub-interfaces start adding more details about the properties of the data structures, likeList
andSet
. -
List: In a
List
, elements are ordered, in a similar fashion as we saw for arrays in the previous section. This means that the objects stored in the list are in a sequence, and we have control over the position/index at which elements are inserted. We can also access objects by their index in the List. Lastly, lists allow duplicates, meaning you can store the same object more than once. -
Set: Sets in Java are modelled after the concept of a set in mathematics. They’re a collection of objects that don’t allow duplicate objects. This interface doesn’t provide a notion of an index for each object.
-
Map: Maps are data structures that allow us to efficiently map
keys
tovalue
objects. For example, think about a dictionary, where eachword
is mapped to one or moremeanings
, where thewords
are the keys and themeanings
are the values associated with each key. The Map interface isn’t a type of Collection as they’re data structures with different purposes.
We’ll go into more detail using the concrete implementations shown in the diagram in the next sections.
It’s important to read the documentation of the actual implementation classes before using them. |